App Deployments
App deployments are currently in preview. If you would like to try them out, please reach out to us
.App deployments are a way to group and publish your GraphQL operations as a single app version to the Hive Registry. This allows you to keep track of your different app versions, their operations usage, and performance.
Making better decisions about:
- Which operations are being used by what app client version (mobile app, web app, etc.)
- Which team or developer is responsible for a specific operation and needs to be contacted for Schema change decissions
Furthermore, it allows you to leverage persisted documents (also knows as trusted documents or persisted queries) on your GraphQL Gateway or server, which provides the following benefits:
- Reduce payload size of your GraphQL requests (reduce client bandwidth usage)
- Secure your GraphQL API by only allowing operations that are known and trusted by your Gateway
Extracting Persisted Documents
We need to extract the persisted documents from within our app. The method for doing this may vary depending on the tools you are using.
The persisted documents must stored in a JSON file, which includes the documents along with their corresponding hashes. This JSON file will be uploaded to the Hive Registry.
{
"d9a00ec0c1ce": "query UsageEstimationQuery($input: UsageEstimationInput!) { __typename usageEstimation(input: $input) { __typename operations } }",
"b8d98e796421": "mutation GenerateStripeLinkMutation($selector: OrganizationSelectorInput!) { __typename generateStripePortalLink(selector: $selector) }"
}
These persisted documents define all the possible operations that your app can execute against your GraphQL API.
As long as the app deployment using these persisted documents is active, any change of the GraphQL API that alters the GraphQL schema as used by any of the persisted documents would break that specific app version and therefore be an unsafe/breaking change.
For GraphQL Code Generator, you can configure the client preset to generate the persisted document manifest.
import { type CodegenConfig } from '@graphql-codegen/cli'
const config: CodegenConfig = {
schema: 'schema.graphql',
documents: ['src/**/*.tsx'],
generates: {
'./src/gql/': {
preset: 'client',
presetConfig: {
persistedDocuments: true
}
}
}
}
After running the Relay Compiler, the file persisted-documents.json
will be generated. This file
can be used for creating a new app deployment on Hive.
For more information, please refer to the GraphQL Code Generator documentation.
Create an App Deployment
To create an app deployment with the extracted persisted documents, you need to have the Hive CLI installed and authenticated with your Hive target, on which you want to deploy your app.
Note: The GraphQL operations being uploaded must pass validation against the target schema. Otherwise, the app deployment creation will fail.
Once the pre-requisites are met, you can create an app deployment by running the following command.
hive app:create \
--registry.accessToken "<ACCESS_TOKEN>" \
--target "<ORGANIZATION>/<PROJECT>/<TARGET>" \
--name "my-app" \
--version "1.0.0" \
persisted-documents.json
The parameters --name
and --version
are mandatory and uniquily identify your app deployment.
Parameter | Description |
---|---|
--name | The name of the app deployment. |
--version | The version of the app deployment. |
<persistedDocumentsFile> | The path to the JSON file containing the persisted documents. |
An app deployment is uniquely identified by the combination of the name
and version
parameters
for each target.
After creating an app deployment, it is in the pending state. That means you can view it on the Hive App UI.
Navigate to the Target page on the Hive Dashboard and click on the Apps tab to see it.
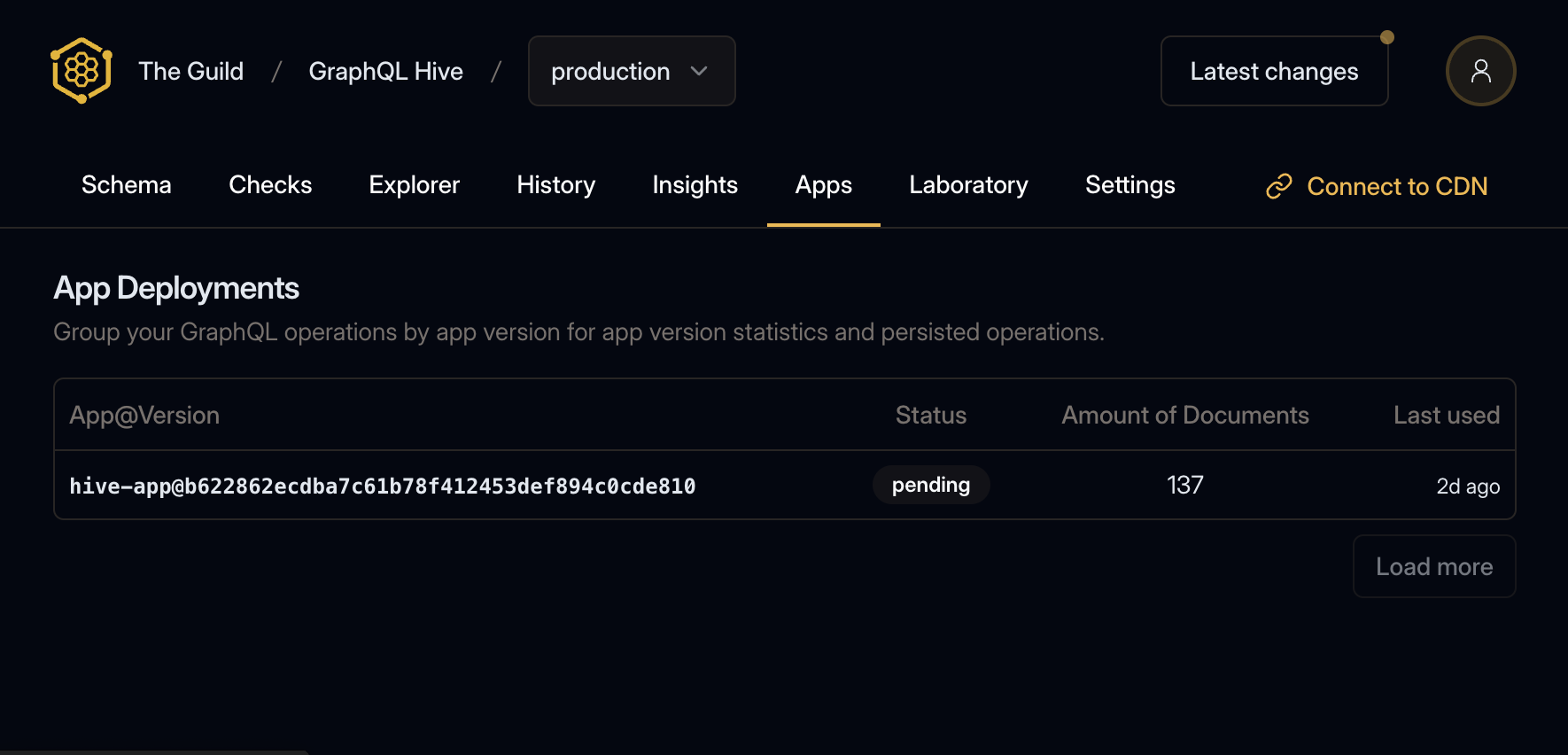
By clicking on the app deployments name, you can navigate to the app deployment details page, where you can see all the GraphQL operations that are uploaded as part of this app deployment.
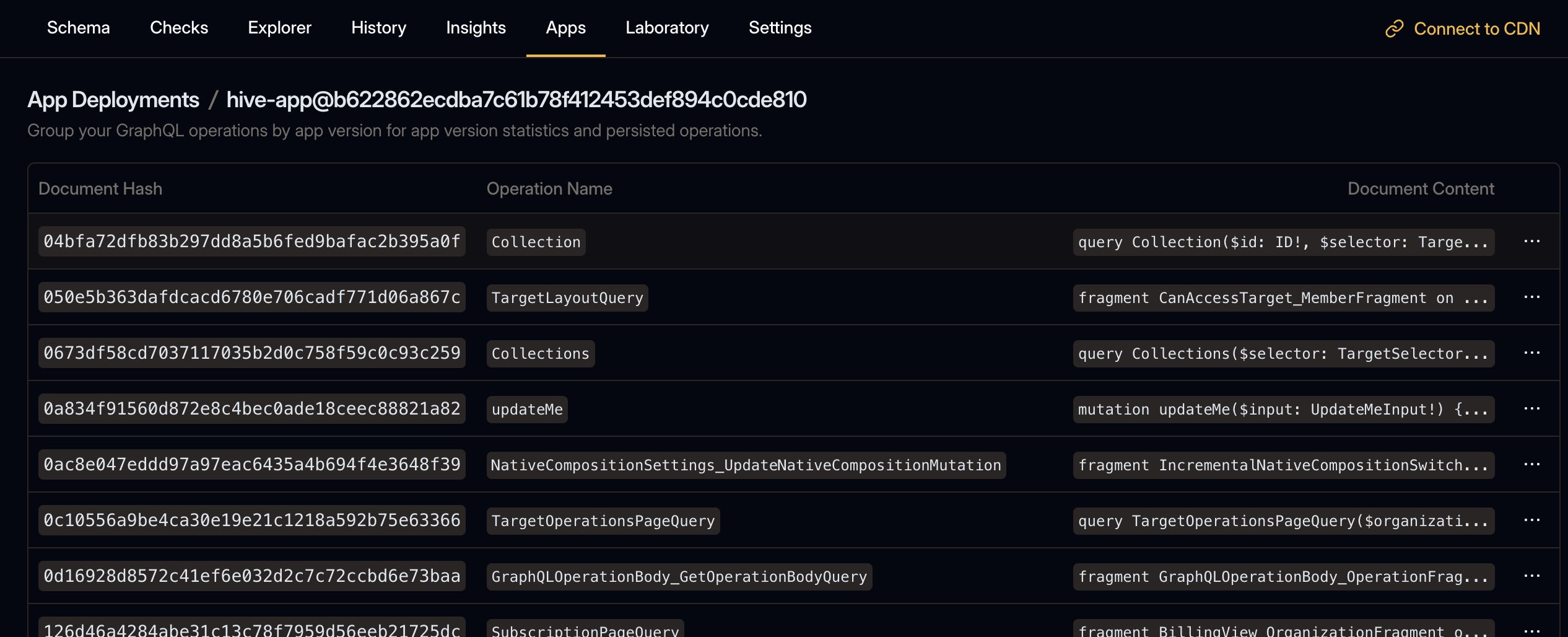
Publish an App Deployment
A app deployment will be in the pending state until you publish it. By publishing the app deployment, the persisted documents will be available via the Hive CDN and can be used by your Gateway or GraphQL API for serving persisted documents to your app.
To publish an app deployment, you can run the following command. Make sure you utilize the same
--name
and --version
parameters as you used when creating the app deployment.
hive app:publish \
--registry.accessToken "<ACCESS_TOKEN>" \
--target "<ORGANIZATION>/<PROJECT>/<TARGET>" \
--name "my-app" \
--version "1.0.0"
Parameter | Description |
---|---|
--name | The name of the app deployment. |
--version | The version of the app deployment. |
After publishing the app deployment, the persisted documents, the status of the app deployment on the Hive dashboard will change to active.
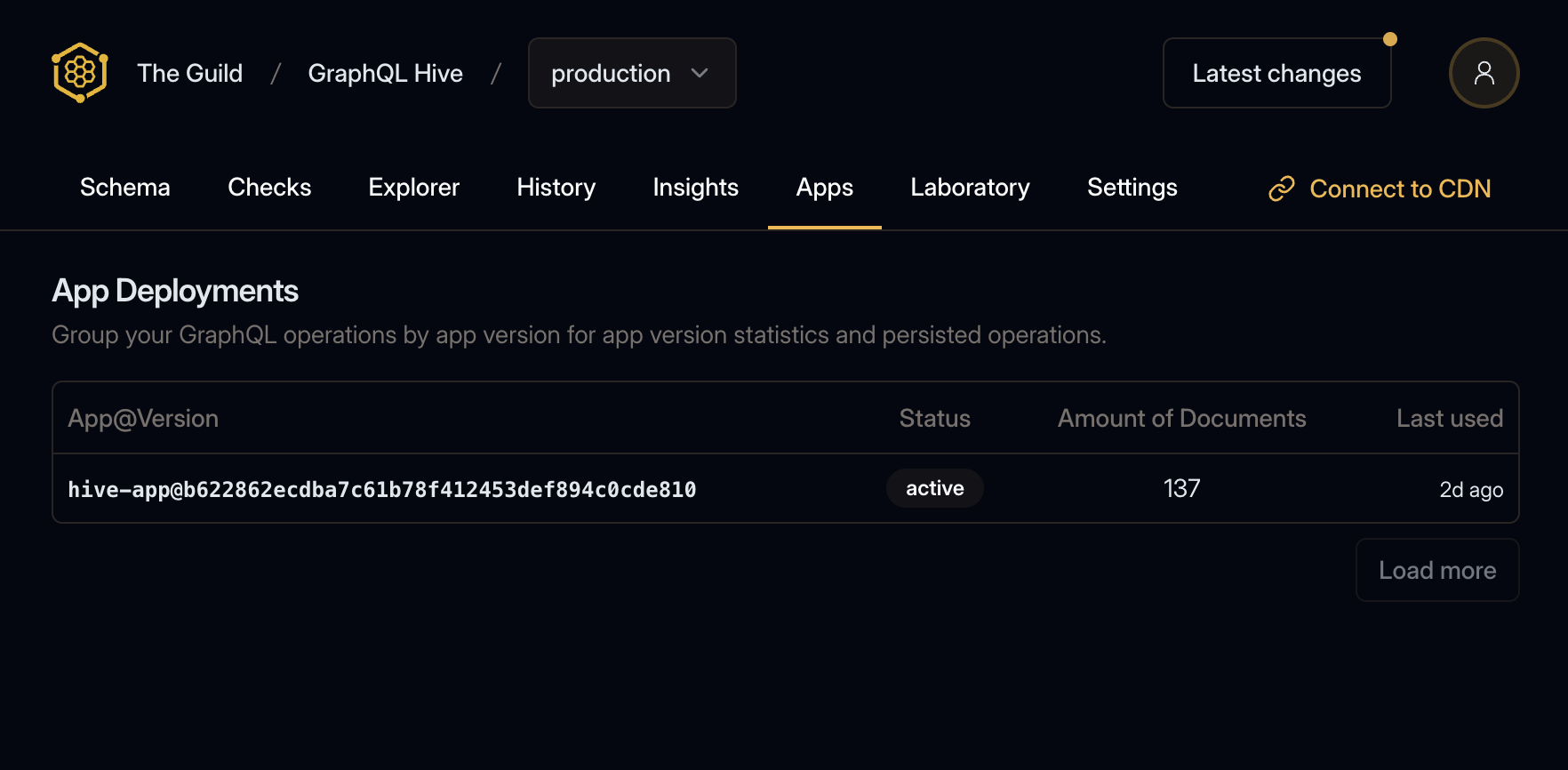
Persisted Documents on GraphQL Server and Gateway
Persisted documents can be used on your GraphQL server or Gateway to reduce the payload size of your GraphQL requests and secure your GraphQL API by only allowing operations that are known and trusted by your Gateway.
Hive serves as the source of truth for the allowed persisted documents and provides a CDN for fetching these documents as they are requested.
For Hive Gateway you can use the Hive configuration for resolving persisted documents. Adjust your
gateway.config.ts
file as follows.
import { defineConfig } from '@graphql-hive/gateway'
export const gatewayConfig = defineConfig({
persistedDocuments: {
type: 'hive',
// The endpoint of Hive's CDN
endpoint: '<supergraph endpoint>',
// The CDN token provided by Hive Registry
key: '<cdn access token>'
}
})
For further information, please refer to the Hive Gateway documentation for persisted documents.
Sending Persisted Document Requests from your App
Once you have the persisted documents available on your GraphQL server or Gateway, you can send a GraphQL request with the persisted document ID following the GraphQL over HTTP specification.
POST /graphql
Content-Type: application/json
{
"documentId": "<app_name>~<app_version>~<document_hash>",
}
Parameter | Description |
---|---|
app_name | The name of the app deployment. |
app_version | The version of the app deployment. |
document_hash | The hash of the persisted document. |
Example curl request:
curl \
-X POST \
-H 'Content-Type: application/json' \
-d '{"documentId": "<app_name>~<app_version>~<document_hash>"}' \
http://localhost:4000/graphql
Continous Deployment (CD) Integration
We recommend integrating the app deployment creation and publishing into your CD pipeline for automating the creationg and publishing of app deployments.
Usually the following steps are performed.
Since the application will be configured to send requests with the persisted document ID, we need to ensure that these documents are available on the Hive CDN before deploying the application.
Extracting the persisted documents usually happens during the build step of your application. E.g. if you are using GraphQL Code Generator with the client preset or Relay, the persisted document JSON mapping is generated as an artifact.
In your CLI pipeline, you can then use the Hive CLI (or Hive CLI docker container) to create and publish the app deployment.
After that, you can deploy your application with confidence.
Tools like GitHub Actions, Pulumni, or Terraform can be used to model this flow. We use it ourselves for deploying the GraphQL Hive Dashboard using Pulumni.